![[Ubuntu] ssh 원격접속 보안 강화](https://blog.kakaocdn.net/dn/vOZdu/btsLzqF56aG/NTpSpB9fXfkkt4pbYiRsTk/img.jpg)
![[DB 성능 최적화] 인덱스(Index)](https://blog.kakaocdn.net/dn/nA2td/btsI9RE76dJ/L4bSgASjdtfHqK9KGAAC4k/img.png)
![[JPA] 병합(Merge)과 변경 감지(Dirty Checking)](https://blog.kakaocdn.net/dn/bFZDNu/btsIWaxR2k8/cSvhbm9EekSz6ubAKDeTkK/img.png)
![[Linux] 프로세스와 시그널](https://blog.kakaocdn.net/dn/bkg05L/btsKDFCOXxP/e8zaWDdfLamdv5yM8KsPZ1/img.jpg)
![[Docker] Docker Compose를 이용한 SpringBoot + MySQL + Redis 컨테이너 동시에 띄우기](https://blog.kakaocdn.net/dn/bQ4rTl/btsJfoCTWhU/Cfi1RaJSboO8ccgtmJepX1/img.webp)
![[Github Actions] 기본 문법 + 스프링부트 프로젝트 배포 자동화](https://blog.kakaocdn.net/dn/PPr6T/btsJtKAsSo9/rdlgK2vLTiqH2KUkdRBqkK/img.png)
![[Database] 트랜잭션 ACID, DB 연결구조와 세션](https://blog.kakaocdn.net/dn/c7kA33/btsFcQKD9PM/TAAAfUh62KENKRsO32rye0/img.png)
![[JAVA] 상속 - 타입 변환과 다형성](https://blog.kakaocdn.net/dn/viEHP/btrVjnz9GER/zaV6B2QF28VWdHsPVapgZ1/img.jpg)
![[JSP] IntelliJ 에서 JSP 개발환경 만들기](https://blog.kakaocdn.net/dn/cNQOvx/btsC7qtoxqm/UuNX5TxNKYHXQ3Wk18KFyk/img.png)
![[Git] 소스트리(Sourcetree)로 브랜치(Branch) 다루기](https://blog.kakaocdn.net/dn/1Ulru/btsCy6Do1i6/E5Ooi4C792QgEUJrdGQKAk/img.png)
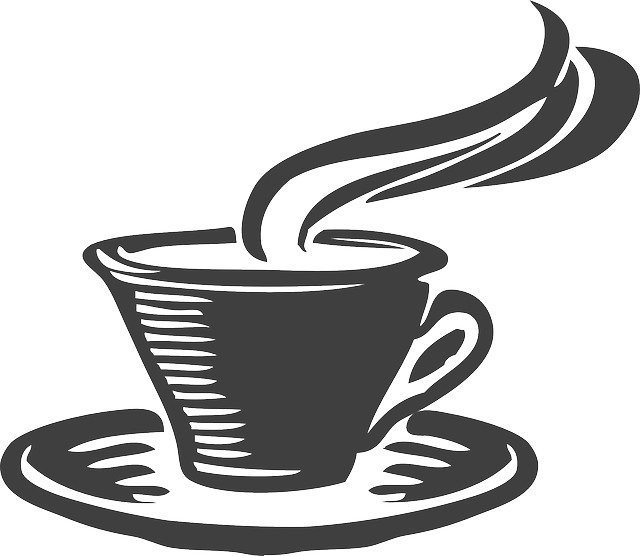
![[GitHub] 소스트리로 clone, push, fetch, pull, pull request 하기](https://blog.kakaocdn.net/dn/b9E4q2/btsCOAoPpDi/FinGvyWAY6SIjfQbXnGhIk/img.png)
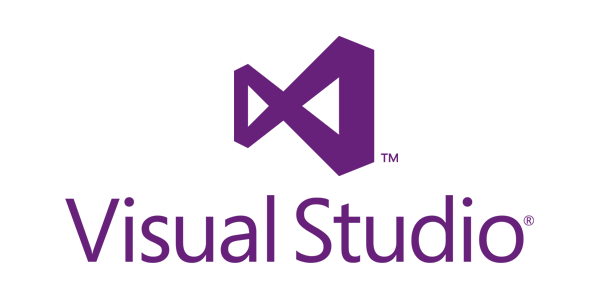
![[Spring] GeoIP를 이용한 해외 IP 차단](https://blog.kakaocdn.net/dn/stShJ/btsLO6109qd/5ZvcbygO69oocyi0UFzNvK/img.png)
MaxMind 에서 데이터베이스 다운로드MaxMind에서 먼저 데이터베이스를 받아와야한다.국가를 제외한 나머지 자료(시, 도 등)는 꽤 부정확하다는 글이 많고, 해외인지 아닌지가 가장 중요하기 때문에 country 데이터베이스만 사용하기로 했다.아래 사이트에서 회원 가입을 한 후 country 데이터베이스를 다운로드 받는다.(GeoLite2-Country.mmdb)https://www.maxmind.com/en/home 스프링에 적용의존성 추가build.gradle에 geoip 의존성을 추가해준다.implementation "com.maxmind.geoip2:geoip2:4.1.0" 서비스 로직 작성아래 서비스 계층에는getClientIP() : request 객체를 바탕으로 IP를 추출하는 메서드 ge..
![[GitHub Actions] Self-Hosted Runner](https://blog.kakaocdn.net/dn/5i0CT/btsLICTlGjx/bW6ihal4usEB0Z5TYKz6I0/img.png)
self-hosted runner를 사용한 이유는 깃허브에서 제공하는 러너를 사용하면 ssh키를 입력하여 기본 제공 러너가 내 서버로 원격 접속해서 배포를 하게 되는데, 나는 내 서버의 ssh키를 누구에게도 알려주고 싶지 않았기 때문에 이를 사용하게 되었다.self-host runner 설치자신의 깃허브 계정에서 원하는 레포지토리의 setting 탭의 Actions -> Runners에 들어간다.설정하고자 하는 로컬 머신에 맞는 이미지와 아키텍쳐를 선택한다.나는 N100 기반의 머신이기 때문에 x64를 선택하였다. 선택하면 아래와 같이 친절하게 명령어가 주어진다.Self-hosted Runner로 등록하고자 하는 기기에서 폴더 생성mkdir actions-runner && cd actions-runne..
![[Ubuntu] GeoIP 를 이용한 해외 IP SSH 접속 차단](https://blog.kakaocdn.net/dn/nqh8H/btsLBJF2AKv/wdaDttjz3HPkB1NChFWl31/img.jpg)
2024.11.29 - [서버 구축기] - [Ubuntu] ssh 원격접속 보안 강화 [Ubuntu] ssh 원격접속 보안 강화최근에 개인 서버를 마련했다. 이 서버에 내 개인 프로젝트를 구동시킬 예정인데, 보안이 신경쓰여 내가할 수 있는 방법을 최대한 써서 보안을 강화하려고 한다. 아래의 내용을 따라하면 다음til.seungwook.com위 글에서 uwf, fail2ban 등 기본적인 보안 설정을 마쳤습니다. ssh포트로 접속하는 요청이 해외에서 들어올 때 이를 차단을 하면 보안이 더 향상될 것이라고 생각을 하였습니다.1차적으로 해외 아이피를 차단하고, 2차적으로 fail2ban을 통해서 한국 아이피로 지속적인 접속 요청을 차단하는 방식을 생각하였습니다. 기본 설치 및 세팅설치geoip-bin과 geo..
![[Nginx] Nginx 기본 보안 설정](https://blog.kakaocdn.net/dn/WqiUq/btsLBW4xDlM/KZxqQ99cdjKlhe7sOysL31/img.png)
먼저/etc/nginx/nginx.conf : Nginx의 메인 설정 파일이며, 글로벌 설정 및 기본 동작 방식을 설정하기 위해 사용/etc/nginx/sites-enabled/default : 개별 서버 블록(사이트) 설정 파일이다. 특정 사이트나 도메인에 대한 설정을 정의이 두 파일의 차이점을 알고 가야한다. 또한 필자는 인증서를 Certbot을 통해서 발급 받았다. 메인 설정 파일 수정sudo nano /etc/nginx/nginx.conf http블록 안에 아래의 내용을 추가한다.(이미 정의되어 있다면 변경한다.)# Nginx 버전정보 노출 끔server_tokens off;# SSL Settingsssl_protocols TLSv1.2 TLSv1.3; # SSL/TLS 인증은 TLSv1, TLS..
![[MySQL] MySQL 보안 설정](https://blog.kakaocdn.net/dn/DxHPH/btsLBdMoFTD/B4KIZcraYhwiKmRntkfS7k/img.png)
서버 환경 : Ubuntu 24.04 LTSMySQL 버전 : 8.0.40 MySQL 설치설치MySQL을 먼저 설치한다.sudo apt update && sudo apt upgrade -ysudo apt install mysql-server -y 설치된 버전을 확인하여 정상적으로 설치되었는지 확인한다.mysql --version나는 위 명령어를 실행했을 때 mysql Ver 8.0.40-0ubuntu0.24.04.1 for Linux on x86_64 ((Ubuntu)) 이 결과가 나왔다. 보안 스크립트 설정보안을 강화하기 위해 아래의 보안 스크립트를 실행한다.sudo mysql_secure_installation Securing the MySQL server deployment. Connecting ..
![[Ubuntu] 외장 SSD ext4 초기화 + 마운트](https://blog.kakaocdn.net/dn/bZjzfH/btsLBxwWoC9/kWDMJo0A6lAcXXZn9KNeJ0/img.jpg)
먼저 SSD를 연결한 후, 디스크가 인식되었는지 확인한다.lsblksda: 477GB 디스크(내부 디스크)sda1: /boot/efi에 마운트된 1GB 파티션.sda2: 루트 파일 시스템(/)에 마운트된 476GB 파티션.sdb: 931GB 디스크 (외장 SSD)sdb1: 전체 디스크 크기를 사용하는 단일 파티션. ext4로 포맷현재 내 외장 ssd는 exFAT으로 포매팅되어있다. 아래의 명령어로 이를 확인할 수 있다.sudo blkid /dev/sdb1 또한 마운트가 되어있다면 마운트를 해제해야 하지만, 마운트를 한적이 없기 때문에 그대로 포맷을 진행한다. mkfs.ext4 명령어를 통해 포맷을 진행한다.server-ssd는 디스크 라벨을 지정한 것이고, dev/sdb1는 현재 연결된 외장 SSD 파티..
나는 PostgreSQL을 homebrew를 통해서 설치하였다. nano /opt/homebrew/var/postgresql@14/pg_hba.conf이 파일을 열면 아래 부분에 아래와 같이 기본적으로 설정되어있다.# TYPE DATABASE USER ADDRESS METHOD# "local" is for Unix domain socket connections onlylocal all all trust# IPv4 local connections:host all all 127.0.0.1/32 ..
![[Ubuntu] ssh 원격접속 보안 강화](https://blog.kakaocdn.net/dn/vOZdu/btsLzqF56aG/NTpSpB9fXfkkt4pbYiRsTk/img.jpg)
최근에 개인 서버를 마련했다. 이 서버에 내 개인 프로젝트를 구동시킬 예정인데, 보안이 신경쓰여 내가할 수 있는 방법을 최대한 써서 보안을 강화하려고 한다. 아래의 내용을 따라하면 다음과 같은 보안적인 이점이 있다.SSH 포트번호 변경root 계정 SSH 접속 비허용RSA를 이용한 공개키 기반 인증허용된 포트만 열어둠(UFW)비정상적인 접근 차단 및 로깅(fail2ban)SSH 2단계 인증(2FA) 서버 환경은 Ubuntu 24.04 LTS 버전에서 이뤄졌다는점 참고 바랍니다. SSH 포트번호 변경ssh 설정파일을 변경해서 포트번호를 바꾸어햔다.sudo nano /etc/ssh/sshd_configPort 부분에 주석이되어있을 텐데 주석을 해제하고 원하는 포트번호로 바꾼다. sudo nano /lib/s..
개발 환경SpringBoot 3.3.5Java 17PostgreSQL 14 문제 상황ERROR: column "XXX" is of type XXX but expression is of type character varying Hint: You will need to rewrite or cast the expression. @Enumerated(EnumType.STRING)@Column(nullable = false, name = "level")private UserLevel level; 엔티티에 ENUM 타입이 있는데 이를 enum이 아닌 문자열로 인식해서 발생하는 문제임을 알았다.XXX(예시) 라는 컬럼이 있는데, PostgreSQL에서는 ENUM으로 만들었지만, JPA에서 이를 문자열로 인식한 상..
![[Bash 스크립트] Bash 스크립트 기본 개념과 변수](https://blog.kakaocdn.net/dn/lg8Gs/btsKDfFg3KO/N9lWkexVwAIdJVkUtBUrIK/img.jpg)
Bash 스크립트 기본 개념리눅스에는 여러가지 쉘이 존재하지만 대부분의 리눅스 배포판에서 Bash가 기본 쉘로 제공되고 있다.사실상 리눅스 쉘의 표준이 Bash이다. Bash의 장단점장점POSIX 표준을 준수해 대부분의 유닉스 기반 시스템에서 일관되게 작동한다. -> 이식성이 좋다.자동 완성, 명령 히스토리, 명령줄 편집 등 사용자 편의 기능이 많아 편리하다.리눅스 배포판 대부분에 기본 쉘로 탑재되어 있어 배우기 쉽고, 관련 문서와 자료도 풍부하다.단점복잡한 작업을 처리할 때 속도나 성능 느리다.일부 구문이 복잡하고 오류가 발생하기 쉬워, 복잡한 스크립트 작성 시 어려움이 있을 수 있다.다른 언어와 달리 객체 지향 기능이 없어, 대규모 프로그램 작성에는 비효율적이다. 동작 방식bash 스크립트는 bash..